February
24th,
2016
目录
iphone沙箱
沙盒文件说明
iphone沙箱模型的有四个文件夹,分别为:
1、Documents 目录:您应该将所有de应用程序数据文件写入到这个目录下。这个目录用于存储用户数据或其它应该定期备份的信息。
2、AppName.app 目录:这是应用程序的程序包目录,包含应用程序的本身。由于应用程序必须经过签名,所以您在运行时不能对这个目录中的内容进行修改,否则可能会使应用程序无法启动。
3、Library 目录:这个目录下有两个子目录:Caches 和 Preferences
Preferences 目录:包含应用程序的偏好设置文件。您不应该直接创建偏好设置文件,而是应该使用NSUserDefaults类来取得和设置应用程序的偏好.
Caches 目录:用于存放应用程序专用的支持文件,保存应用程序再次启动过程中需要的信息。
4、tmp 目录:这个目录用于存放临时文件,保存应用程序再次启动过程中不需要的信息。
获取沙盒几个目录的方式
// 获取沙盒主目录路径
NSString *homeDir = NSHomeDirectory();
// 获取Documents目录路径
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *docDir = [paths objectAtIndex:0];
// 获取Caches目录路径
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES);
NSString *cachesDir = [paths objectAtIndex:0];
// 获取tmp目录路径
NSString *tmpDir = NSTemporaryDirectory();
// 获取当前程序包中一个图片资源(apple.png)路径
NSString *imagePath = [[NSBundle mainBundle] pathForResource:@"apple" ofType:@"png"];
UIImage *appleImage = [[UIImage alloc] initWithContentsOfFile:imagePath];
NSFileManager文件操作封装
NSFileManager:此类主要是对文件进行的操作以及文件信息的获取
获取Documents路径:
- (NSString *)getDocumentsPath
{
//获取Documents路径
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *path = [paths objectAtIndex:0];
NSLog(@"path:%@", path);
return path;
}
创建文件夹
-(void)createDirectory{
NSString *documentsPath =[self getDocumentsPath];
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *iOSDirectory = [documentsPath stringByAppendingPathComponent:@"iOS"];
BOOL isSuccess = [fileManager createDirectoryAtPath:iOSDirectory withIntermediateDirectories:YES attributes:nil error:nil];
if (isSuccess) {
NSLog(@"success");
} else {
NSLog(@"fail");
}
}
创建文件
-(void)createFile{
NSString *documentsPath =[self getDocumentsPath];
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *iOSPath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
BOOL isSuccess = [fileManager createFileAtPath:iOSPath contents:nil attributes:nil];
if (isSuccess) {
NSLog(@"success");
} else {
NSLog(@"fail");
}
}
写文件
-(void)writeFile{
NSString *documentsPath =[self getDocumentsPath];
NSString *iOSPath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
NSString *content = @"我要写数据啦";
BOOL isSuccess = [content writeToFile:iOSPath atomically:YES encoding:NSUTF8StringEncoding error:nil];
if (isSuccess) {
NSLog(@"write success");
} else {
NSLog(@"write fail");
}
}
读取文件内容
-(void)readFileContent{
NSString *documentsPath =[self getDocumentsPath];
NSString *iOSPath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
NSString *content = [NSString stringWithContentsOfFile:iOSPath encoding:NSUTF8StringEncoding error:nil];
NSLog(@"read success: %@",content);
}
判断文件是否存在
- (BOOL)isSxistAtPath:(NSString *)filePath{
NSFileManager *fileManager = [NSFileManager defaultManager];
BOOL isExist = [fileManager fileExistsAtPath:filePath];
return isExist;
}
计算文件大小
- (unsigned long long)fileSizeAtPath:(NSString *)filePath{
NSFileManager *fileManager = [NSFileManager defaultManager];
BOOL isExist = [fileManager fileExistsAtPath:filePath];
if (isExist){
unsigned long long fileSize = [[fileManager attributesOfItemAtPath:filePath error:nil] fileSize];
return fileSize;
} else {
NSLog(@"file is not exist");
return 0;
}
}
计算整个文件夹中所有文件大小
- (unsigned long long)folderSizeAtPath:(NSString*)folderPath{
NSFileManager *fileManager = [NSFileManager defaultManager];
BOOL isExist = [fileManager fileExistsAtPath:folderPath];
if (isExist){
NSEnumerator *childFileEnumerator = [[fileManager subpathsAtPath:folderPath] objectEnumerator];
unsigned long long folderSize = 0;
NSString *fileName = @"";
while ((fileName = [childFileEnumerator nextObject]) != nil){
NSString* fileAbsolutePath = [folderPath stringByAppendingPathComponent:fileName];
folderSize += [self fileSizeAtPath:fileAbsolutePath];
}
return folderSize / (1024.0 * 1024.0);
} else {
NSLog(@"file is not exist");
return 0;
}
}
删除文件
-(void)deleteFile{
NSString *documentsPath =[self getDocumentsPath];
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *iOSPath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
BOOL isSuccess = [fileManager removeItemAtPath:iOSPath error:nil];
if (isSuccess) {
NSLog(@"delete success");
}else{
NSLog(@"delete fail");
}
}
移动文件
- (void)moveFileName
{
NSString *documentsPath =[self getDocumentsPath];
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *filePath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
NSString *moveToPath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
BOOL isSuccess = [fileManager moveItemAtPath:filePath toPath:moveToPath error:nil];
if (isSuccess) {
NSLog(@"rename success");
}else{
NSLog(@"rename fail");
}
}
重命名
- (void)renameFileName
{
//通过移动该文件对文件重命名
NSString *documentsPath =[self getDocumentsPath];
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *filePath = [documentsPath stringByAppendingPathComponent:@"iOS.txt"];
NSString *moveToPath = [documentsPath stringByAppendingPathComponent:@"rename.txt"];
BOOL isSuccess = [fileManager moveItemAtPath:filePath toPath:moveToPath error:nil];
if (isSuccess) {
NSLog(@"rename success");
}else{
NSLog(@"rename fail");
}
}
NSFileHandle 文件操作封装
NSFileHandle:此类主要是对文件内容进行读取和写入操作.
NSFileHandle处理文件的步骤:
-
打开文件,并获取一个NSFileHandle对象,以便在后面的I/O操作中引用该文件
-
对打开的文件执行I/O操作(读取、写入、更新)
-
关闭文件
常用处理方法
+ (id)fileHandleForReadingAtPath:(NSString *)path 打开一个文件准备读取
+ (id)fileHandleForWritingAtPath:(NSString *)path 打开一个文件准备写入
+ (id)fileHandleForUpdatingAtPath:(NSString *)path 打开一个文件准备更新
- (NSData *)availableData; 从设备或通道返回可用的数据
- (NSData *)readDataToEndOfFile; 从当前的节点读取到文件的末尾
- (NSData *)readDataOfLength:(NSUInteger)length; 从当前节点开始读取指定的长度数据
- (void)writeData:(NSData *)data; 写入数据
- (unsigned long long)offsetInFile; 获取当前文件的偏移量
- (void)seekToFileOffset:(unsigned long long)offset; 跳到指定文件的偏移量
- (unsigned long long)seekToEndOfFile; 跳到文件末尾
- (void)truncateFileAtOffset:(unsigned long long)offset; 将文件的长度设为offset字节
- (void)closeFile; 关闭文件
向文件追加数据
- (void)writeDataToFile:(NSData *)aData{
NSString *homePath = NSHomeDirectory( );
NSString *sourcePath = [homePath stringByAppendingPathConmpone:@"testfile.text"];
NSFileHandle *fielHandle = [NSFileHandle fileHandleForUpdatingAtPath:sourcePath];
[fileHandle seekToEndOfFile]; 将节点跳到文件的末尾
[fileHandle writeData:aData]; 追加写入数据
[fileHandle closeFile];
}
复制文件
- (void)copyFromFile:(NSString *)sourcePath toOutPath:(NSString *)outPath{
NSFileHandle *infile, *outfile; //输入文件、输出文件
NSData *buffer; //读取的缓冲数据
NSFileManager *fileManager = [NSFileManager defaultManager];
NSString *homePath = NSHomeDirectory( );
BOOL sucess = [fileManager createFileAtPath:outPath contents:nil attributes:nil];
if (!success)
{
return N0;
}
infile = [NSFileHandle fileHandleForReadingAtPath:sourcePath]; 创建读取源路径文件
if (infile == nil)
{
return NO;
}
outfile = [NSFileHandle fileHandleForReadingAtPath:outPath]; 创建病打开要输出的文件
if (outfile == nil)
{
return NO;
}
[outfile truncateFileAtOffset:0]; 将输出文件的长度设为0
buffer = [infile readDataToEndOfFile]; 读取数据
[outfile writeData:buffer]; 写入输入
[infile closeFile]; 关闭写入、输入文件
[outfile closeFile];
}
向指定文件中读出数据
- (NSData *)readDataFromFile:(NSString *)filepath fromLength:(NSUInteger)length{
NSFileHandle *filehandle = [NSFileHandle fileHandleForUpdatingAtPath:filepath];
NSUInteger length = [filehandle availableData].length;
[filehandle seekToFileOffset:length/2];
NSData *data = [filehandle readDataToEndOfFile];
NSString *str = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
NSLog(@"the data is :%@",str);
[filehandle closeFile];
}
如果觉得我的文章对您有用,请随意打赏。您的支持将鼓励我继续创作!
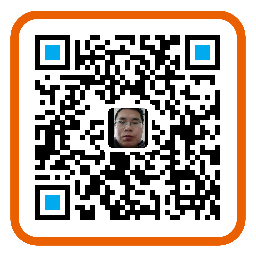